You all must have seen a seven segment display.If not here it is:
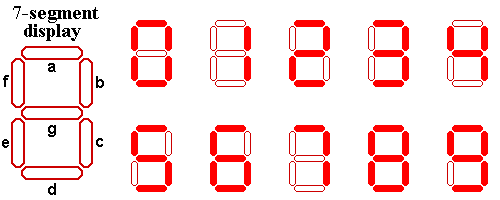
Alice got a number written in seven segment format where each segment was creatted used a matchstick.
Example: If Alice gets a number 123 so basically Alice used 12 matchsticks for this number.
Alice is wondering what is the numerically largest value that she can generate by using at most the matchsticks that she currently possess.Help Alice out by telling her that number.
Input Format:
First line contains T (test cases).
Next T lines contain a Number N.
Output Format:
Print the largest possible number numerically that can be generated using at max that many number of matchsticks which was used to generate N.
In C -
#include<stdio.h>
#include<string.h>
int main(void)
{
int t;
scanf("%d",&t);
while(t--)
{
int a[10]={6,2,5,5,4,5,6,3,7,6,},i,sum=0;
char n[100];
scanf("%s",n);
for(i=0;i<strlen(n);i++)
sum+= a[(n[i]-48)];
if(sum%2==1)
printf("7");
else
printf("1");
for(i=1;i<sum/2;i++)
printf("1");
printf("\n");
}
}
In C++ -
#include<iostream>
#include<cstring>
using namespace std;
int main()
{
int T;
cin >> T;
while(T--){
char arr[100];
cin >> arr;
int sticks = 0;
for(int i=0; i<strlen(arr); i++){
if(arr[i] == '0' || arr[i] == '6' || arr[i] == '9')
sticks += 6;
else if(arr[i] == '1')
sticks += 2;
else if(arr[i] == '2' || arr[i] == '3' || arr[i] == '5')
sticks += 5;
else if(arr[i] == '4')
sticks += 4;
else if(arr[i] == '7')
sticks += 3;
else if(arr[i] == '8')
sticks += 7;
}
if(sticks % 2 == 0){
sticks = sticks / 2;
while(sticks--){
cout << "1";
}
cout << endl;
}
else{
sticks = sticks / 2;
sticks--;
cout << "7";
while(sticks--){
cout << "1";
}
cout << endl;
}
}
return 0;
}
By - Shashank Ravindra
In Java -
import java.io.BufferedReader;
import java.io.InputStreamReader;
class TestClass {
public static void main(String args[] ) throws Exception {
int arr[]=new int[]{6,2,5,5,4,5,6,3,7,6};
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int test=Integer.parseInt(br.readLine());
StringBuilder str=new StringBuilder("");
while(test--!=0)
{
int count=0;
String st=br.readLine();
for(int i=0; i<st.length(); i++)
{
count+=arr[st.charAt(i)-'0'];
}
if(count%2==1)
{
str.append("7");
count-=3;
for(int i=0; i<count; i+=2)
{
str.append("1");
}
}
else
{
for(int i=0; i<count; i+=2)
{
str.append("1");
}
}
str.append("\n");
}
System.out.println(str);
}
}
By - Gaurav
In Python -
def mat(m):
x = 0
match={
"0": 6, "1": 2,"2":5, "3": 5,
"4": 4, "5": 5, "6": 6,
"7": 3, "8": 7,"9": 6 }
for i in m :
x += match[i]
r = x % 2
result = ["1"]
if r == 0 :
result.append(x // 2)
result.append("")
elif r == 1:
result.append((x // 2)-1)
result.append("7")
return ((result[0] * result[1]) + result[2])
n = int(input())
while n!=0:
m = input()
print(mat(m)[::-1])
n = n-1
By - Mk Mourya
Can you pls tel me why you have used n[I]-48
ReplyDeletePost a Comment